Martijn Dull
Read all my blogsImplementing automated Cypress E2E tests in the frontend
In the world of E-commerce, ensuring the quality of your webshop is crucial for success. Your customers expect a fast, reliable, and user-friendly experience when shopping online, and any issues or errors can quickly lead to frustration and lost sales. This is why testing during the development of your webshop is essential. Yet often the client requests that most of the development time is spent on creating new features, since the impression is generally that this adds more value for the customer. This leaves the developers with little time to implement and execute tests to check that the webshop is working as intended.
To make testing easier and save time, it is a good idea to automate this process as much as possible. By automating the tests, you will only have to check the results of the tests instead of executing all the testing steps yourself and when a test fails you can investigate further. It also ensures that tests will be executed in the exact same way every time and should always lead to the same results. So when a new change in the code breaks a part of the site it can be caught easily before it is deployed to production.
Cypress is a popular end-to-end (E2E) testing framework that can be used for this purpose. In this blog I will guide you through an example on how to implement the Cypress E2E testing framework to test the frontend of your webshop.
Getting Started
Before we begin, we need to make sure that we have the necessary tools installed on our machine. In this example we’ll make use of Node.js and NPM.
We can install Cypress by running the following command in our terminal:
npm install cypress --save-dev
Once we have Cypress installed, we can open Cypress by running the following command:
npx cypress open
This will open Cypress and create a new Cypress project in the folder where we ran the command. Cypress will open a new window where we can choose to setup E2E testing. Click on continue and a few example files will be generated to help us get started. We can then choose our preferred browser for testing and a new window with our tests will open.
Creating and running a test
We can create a new test (called “spec” in Cypress), by clicking on “Create new spec”. This will generate the following example:
[abap]
describe(‘template spec’, () => {
it(‘passes’, () => {
cy.visit(‘https://example.cypress.io’)
})
})
[/abap]
We can then test this example by clicking on “run”. This test will pass if it is able to successfully load “https://example.cypress.io”.
We will now change the content of this spec to test a part of our storefront. For this example, we want to create a simple test that tests the following:
- The webshop is available
- The title of the webshop is “My Webshop”
- The homepage displays 12 products
- It is possible to add a product to the cart
To test this, we could rewrite the spec like this:
[abap]
describe(‘Homepage’, () => {
beforeEach(() => {
cy.visit(‘https://myexamplewebshop.com’)
})
it(‘should display the correct title’, () => {
cy.title().should(‘eq’, ‘My Webshop’)
})
it(‘should display the correct number of products’, () => {
cy.get(‘[data-cy="product"]’).should(‘have.length’, 12)
})
it(‘should be possible to add a product to the cart’, () => {
cy.get(‘[data-cy="product-add-to-cart-button"]’).first().click()
cy.get(‘[data-cy="cart-count"]’).should(‘contain’, ‘1’)
})
})
[/abap]
In this example, we have three tests:
- The first test checks that the page title equals “My Webshop”.
- The second test checks that there are 12 products on the homepage.
- The third test checks that it’s possible to add a product to the cart.
We can now run the tests to see if they all pass or if some tests fail and we need to make changes to our code to fix possible bugs.
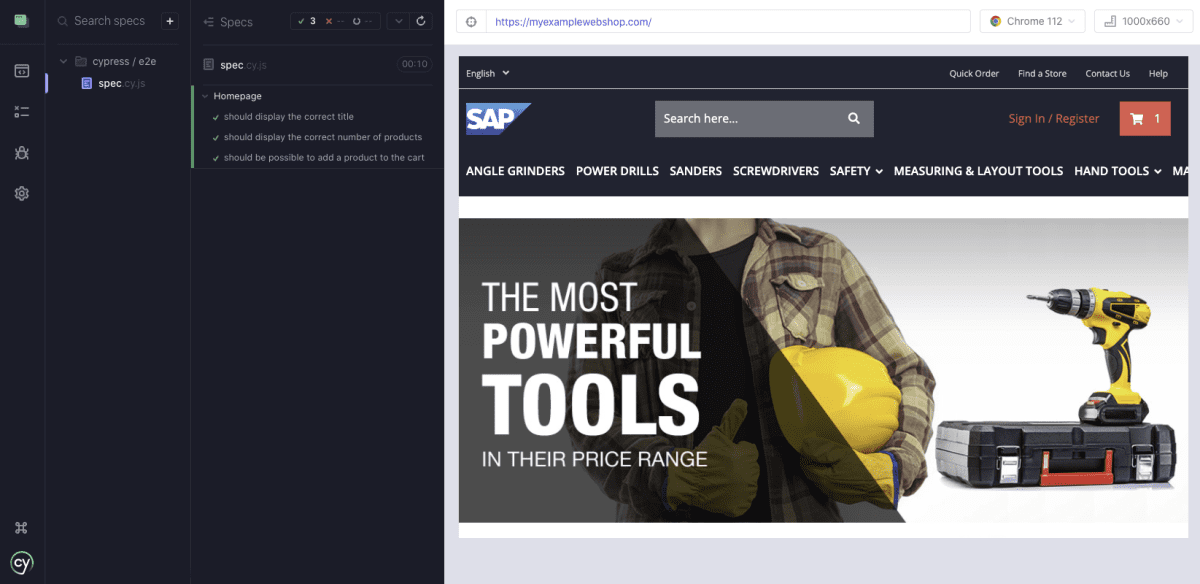
When taking a look at the results after running the tests, we can see that all three of the tests have passed and we can safely bring the code into production.
By running these tests every time changes to the storefront are made, we can easily check if the changes broke any of the existing functionality without having to manually go through the webshop ourselves.
Next steps
I hope this small example helps you get started on implementing tests on your own website so you can reduce the amount of time you spend on testing and give you more time to spend on developing new features.
If you would like to learn more about E2E testing with the Cypress testing framework, you can read the official documentation which includes examples and best practices to help you on your way. The official documentation can be found here.